
import numpy as np
import matplotlib.pyplot as plt
x0 = 0.0
y0 = 1.0
#----- derivative coefficient ----
d_y1 = -np.sin(3*x0) - 3*y0
d_y2 = -3*np.cos(3*x0) - 3*d_y1
d_y3 = 3**2*np.sin(3*x0) - 3*d_y2
d_y4 = 3**3*np.cos(3*x0) - 3*d_y3
d_y5 = -3**4*np.sin(3*x0) - 3*d_y4
d_y6 = -3**5*np.cos(3*x0) - 3*d_y5
d_y7 = 3**6*np.sin(3*x0) - 3*d_y6
d_y8 = 3**7*np.cos(3*x0) - 3*d_y7
d_y9 = -3**8*np.sin(3*x0) - 3*d_y8
n = np.arange(0, 41)
x = n/40
h=1/40
#----- precision solution ---
y = (np.cos(3*x) -np.sin(3*x) + 5*np.e**(-3*x))/6
#----- Numerical solution ---
y2 = y0 + (n*h)*d_y1
y5 = y0 + (n*h)*d_y1 + (n*h)**2 * (d_y2/2) + (n*h)**3 * (d_y3/6) + (n*h)**4 * (d_y4/24)
y10 = y0 + (n*h)*d_y1 + (n*h)**2 * (d_y2/2) + (n*h)**3 * (d_y3/6) + (n*h)**4 * (d_y4/24) + (n*h)**5 * (d_y5/120) + (n*h)**6 * (d_y6/720) + (n*h)**7 * (d_y7/5040) + (n*h)**8 * (d_y8/40320) + (n*h)**9 * (d_y9/362880)
print(" x y y2 y5 y10")
for i in n:
print("%6.3f%14.8f%14.8f%14.8f%14.8f" % (x[i], y[i], y2[i], y5[i],y10[i]))
plt.title("Ordinary Differential Equation")
plt.xlabel("x")
plt.ylabel("y")
plt.grid(True)
plt.plot(x, y, label='precision solution')
plt.scatter(x, y2, marker='0', label='Up to item 2')
plt.scatter(x, y5, marker='^', label='Up to item 5')
plt.scatter(x, y10, marker='s', label='Up to item 2')
plt.xlim(0,0.9, 0.1)
plt.ylim(-0.4, 1.2, 0.2)
plt.legend()
plt.show()
Traceback (most recent call last):
File "/usr/local/lib/python3.7/site-packages/matplotlib/markers.py", line 305, in set_marker
Path(marker)
File "/usr/local/lib/python3.7/site-packages/matplotlib/path.py", line 132, in __init__
cbook._check_shape((None, 2), vertices=vertices)
File "/usr/local/lib/python3.7/site-packages/matplotlib/cbook/__init__.py", line 2304, in _check_shape
f"{k!r} must be {len(target_shape)}D "
ValueError: 'vertices' must be 2D with shape (M, 2). Your input has shape ().
The above exception was the direct cause of the following exception:
Traceback (most recent call last):
File "exper11_2.py", line 35, in <module>
plt.scatter(x, y2, marker='0', label='Up to item 2')
File "/usr/local/lib/python3.7/site-packages/matplotlib/pyplot.py", line 2895, in scatter
**({"data": data} if data is not None else {}), **kwargs)
File "/usr/local/lib/python3.7/site-packages/matplotlib/__init__.py", line 1447, in inner
return func(ax, *map(sanitize_sequence, args), **kwargs)
File "/usr/local/lib/python3.7/site-packages/matplotlib/cbook/deprecation.py", line 411, in wrapper
return func(*inner_args, **inner_kwargs)
File "/usr/local/lib/python3.7/site-packages/matplotlib/axes/_axes.py", line 4473, in scatter
marker_obj = mmarkers.MarkerStyle(marker)
File "/usr/local/lib/python3.7/site-packages/matplotlib/markers.py", line 228, in __init__
self.set_marker(marker)
File "/usr/local/lib/python3.7/site-packages/matplotlib/markers.py", line 309, in set_marker
.format(marker)) from err
ValueError: Unrecognized marker style '0'
エラー原因がわからないです
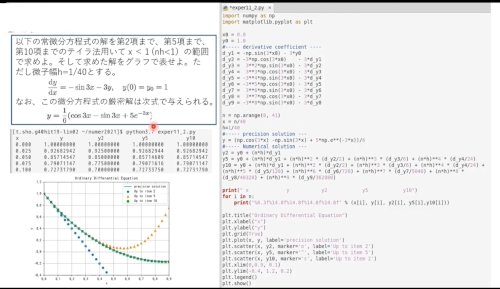
No.1
- 回答日時:
ぱっと見、問ま題が2つ
①マーカーに '0' は指定できない。'o' のつもりでは?
② xlim, ylim はパラメータ2個の筈(matplotlib 3.6)。
Call signatures::
left, right = xlim() # return the current xlim
xlim((left, right)) # set the xlim to left, right
xlim(left, right) # set the xlim to left, right
bottom, top = ylim() # return the current ylim
ylim((bottom, top)) # set the ylim to bottom, top
ylim(bottom, top) # set the ylim to bottom, top
お探しのQ&Aが見つからない時は、教えて!gooで質問しましょう!
似たような質問が見つかりました
- Ruby 教えてください 2 2023/01/04 17:50
- その他(プログラミング・Web制作) python flask から fastapiへの移行時のエラー対処 1 2023/02/05 12:26
- その他(プログラミング・Web制作) pythonをjupiter notebookからmecabで頻出の高い単語の抽出について(Runt 1 2022/12/17 18:08
- その他(プログラミング・Web制作) Pythonで会員サイトの自動ログイン ID Nameがない 1 2022/12/16 02:09
- Ruby パイソンエラーについて 1 2022/12/24 14:07
- その他(プログラミング・Web制作) ラズパイ上の、pythonのエラーについて 1 2023/04/12 23:27
- その他(プログラミング・Web制作) ColabでのPytorchのエラー 1 2022/11/19 20:51
- サーバー WindowsでApache が起動しない 1 2022/11/29 12:21
- Ruby pythonエラー 4 2022/11/11 19:12
- その他(プログラミング・Web制作) pythonのこのエラーがわかりません 3 2022/11/16 14:54
関連するカテゴリからQ&Aを探す
おすすめ情報
- ・漫画をレンタルでお得に読める!
- ・一番好きなみそ汁の具材は?
- ・泣きながら食べたご飯の思い出
- ・「これはヤバかったな」という遅刻エピソード
- ・初めて自分の家と他人の家が違う、と意識した時
- ・いちばん失敗した人決定戦
- ・思い出すきっかけは 音楽?におい?景色?
- ・あなたなりのストレス発散方法を教えてください!
- ・もし10億円当たったら何に使いますか?
- ・何回やってもうまくいかないことは?
- ・今年はじめたいことは?
- ・あなたの人生で一番ピンチに陥った瞬間は?
- ・初めて見た映画を教えてください!
- ・今の日本に期待することはなんですか?
- ・集中するためにやっていること
- ・テレビやラジオに出たことがある人、いますか?
- ・【お題】斜め上を行くスキー場にありがちなこと
- ・人生でいちばんスベッた瞬間
- ・コーピングについて教えてください
- ・あなたの「プチ贅沢」はなんですか?
- ・コンビニでおにぎりを買うときのスタメンはどの具?
- ・おすすめの美術館・博物館、教えてください!
- ・【お題】大変な警告
- ・洋服何着持ってますか?
- ・みんなの【マイ・ベスト積読2024】を教えてください。
- ・「これいらなくない?」という慣習、教えてください
- ・今から楽しみな予定はありますか?
- ・AIツールの活用方法を教えて
- ・最強の防寒、あったか術を教えてください!
- ・歳とったな〜〜と思ったことは?
- ・モテ期を経験した方いらっしゃいますか?
- ・好きな人を振り向かせるためにしたこと
- ・スマホに会話を聞かれているな!?と思ったことありますか?
- ・それもChatGPT!?と驚いた使用方法を教えてください
- ・見学に行くとしたら【天国】と【地獄】どっち?
- ・これまでで一番「情けなかったとき」はいつですか?
- ・この人頭いいなと思ったエピソード
- ・あなたの「必」の書き順を教えてください
- ・14歳の自分に衝撃の事実を告げてください
- ・人生最悪の忘れ物
- ・あなたの習慣について教えてください!!
デイリーランキングこのカテゴリの人気デイリーQ&Aランキング
-
perl DBD::Pg インストールでエ...
-
DBIをCPANからインストール時に...
-
python 環境構築について
-
例外処理のフローチャートの記...
-
Excel VBAでリンク切れをチェッ...
-
エクセルVBAでシートモジュール...
-
「デバイスは PRN を初期化でき...
-
Excel VBA 『Call』で呼び出す...
-
Excel VBAで、ユーザーフォーム...
-
vba userFormのSubを標準モジュ...
-
ExcelでTelnetを動かしたい
-
Perl モジュールのアンインスト...
-
教えて下さい。
-
VBSがコンパイルエラーになりま...
-
vba 標準モジュールインポート...
-
ユーザー定義関数に#NAME?が返...
-
Access VBA標準モジュールにつ...
-
プロキシ経由でjavamailを使っ...
-
VB.NETでの他アプリケーション...
-
大量の標準モジュールを解放す...
マンスリーランキングこのカテゴリの人気マンスリーQ&Aランキング
-
python 環境構築について
-
@INCにrequireされたファイルが...
-
SOAP::Liteを利用したい!
-
Net::SSH::Perlについて
-
DBIをCPANからインストール時に...
-
Pg.pmモジュールがロードできない
-
perl DBD::Pg インストールでエ...
-
YAMLについて
-
perlのモジュールについて
-
ワーニング? encoding.pm
-
Python エクセル読み込み xlrd...
-
'cgi-lib.pl'などのファイルの...
-
Jcode.pmについて
-
python
-
CygwinでPerlのCSV_XSを実行する
-
Perl+DBD::Oracleのエラーがわ...
-
デバッガでブレークポイントを...
-
XML::Parser::Expat が無い?
-
use lib qw(変数名);は無理?
-
LibXMLのエラーについて
おすすめ情報